Components¶
Below are the set of built-in components. Additional custom components are available
via custom components
draw¶
The draw component provides the basic drawing primitives to make shapes. The draw commands mostly correspond to svg path commands, but there are some useful additions.
Draw component should be in the form:
{
"id": "my_drawing",
"type": "draw",
"commands": [
{
"command": "move",
"to": "2,2"
}
]
}
Commands¶
move
Move the cursor to this positionto
Point where to move to
rel_move
Move, with relative position. This means that if the current position is3,4 and
to
is 1,1 then the final position will be 4,5.to
Relative point
line
Draw a line to the requested pointto
Point to draw a line to
rel_line
Same as line only using relative coordsto
relative point
line_by_angle
Draw a line from current position by an angle and lengthangle
angle in degreeslength
length of the line
curve
Draw a cubic bezier curve.to
ctrl_start
ctrl_end
rel_curve
Same as curve, but all points are relativesmooth_curve
A curve where the start control point is reflected from theend control point of the previous curve
to
ctrl_end
rel_smooth_curve
Same assmooth_curve
with points relativecircle
Draw a circleradius
rectangle
Draw a rectanglewidth
height
round_corner
Draws a 90 degree rounded cornerto
The endpoint of the cornerradius
the radius of the curvecorner
The point where the corner would appear
rel_round_corner
svg
Draws an svg ‘Path’svg
The svg path string, (ex: “M 2,2 L 0,0”).
svg_scale_to
Similiar tosvg
command. This is a special draw command which scalesthe svg x and y so that the start point aligns with the previous endpoint and the endpoint aligns to ‘to’. Note, this does not rotate the shape, it only scales in x and y.
svg
The svg path string, (ex: “M 2,2 L 0,0”).to
Where to draw the svg to.reverse
if true this will reverse the path
rel_svg_scale_to
Samce assvg_scale_to
butto
is using relative coodrdinatessvg_connect_to
Similiar tosvg_scale_to
command. This is a special draw command which scalesand rotates the svg x and y so that the start point aligns with the previous endpoint and the endpoint aligns to ‘to’.
svg
The svg path string, (ex: “M 2,2 L 0,0”).to
Where to draw the svg to.svg_from
The starting point in the SVG to use as the connection point. Bydefault this is the start of the path
svg_to
The end point in the SVG to use as the connection point. Bydefault this is the end of the path
reverse
if true this will reverse the path. Default is false
rel_svg_connect_to
Same assvg_connect_to
butto
is using relative coodrdinates
basic_edge¶
an edge is some form of a connection from x1,y1 to x2,y2.
Typically an edge will be written to be reused as a custom component, where the to
and from
params are
passed in.
Parameters¶
handle
: When moving the edge what point should be considered the start. Defaultsto $ORIGIN
to
: The point the edge should be drawn toThe to point can take the following forms:
x, y
{ "x": 5, "y": 6 }
{ "angle" : 90, "length" : 5 } // angle is in degrees where 0 is a straight line to // the right, and 90 is a line pointing to positive Y
from
: The point the edge should be drawn from. Defaults to current positionedge_variable_name
: This is a special feature, if you set this name then certain Attributes of this edge will be available to all subsequently rendered components. See Global Variables
Global Variables¶
<edge_variable_name>__length
: length of this edge<edge_variable_name>__angle
: the angle of this edge
repeat_edge¶
Examples
an edge is some form of a connection from x1,y1 to x2,y2. Edges are specifically designed to be useful for joints, but can easily be used for other things.
The repeat edge is a special variety of edge, that contains some repeatable element. Each repeat edge contains three subcomponents left, repeatable, right. (i.e. beginning, middle, end). Each of the subcomponents should be drawn horizontally from origin 0,0. The component will stitch them together to make a continuous edge, and handle automatically handle moving and rotating.
Typically an edge will be written to be reused, where the to
and from
params are
passed in.
Parameters¶
padding_left
: Additional amount that should be used on the left side beforethe repeatable starts
padding_right
: seepadding_left
handle
: When moving the edge what point should be considered the start. Defaultsto $ORIGIN
to
: The point the edge should be drawn toThe to point can take the following forms:
x, y
{ "x": 5, "y": 6 }
{ "angle" : 90, "length" : 5 } // angle is in degrees where 0 is a straight line to // the right, and 90 is a line pointing to positive Y
from
: The point the edge should be drawn from. Defaults to current positionleft
: This should be a renderable component. it is the left most part of the edge.this should be stretchable and contains a special param called
left_width
.
repeatable
: This should be a renderable component. it is the middle section, which will be repeated as many times as neededright
: Same as left, but hasright_width
special paramedge_variable_name
: This is a special feature, if you set this name then certain Attributes of this edge will be available to all subsequently rendered components. See Global Variables
Global Variables¶
<edge_variable_name>__length
: length of this edge<edge_variable_name>__angle
: the angle of this edge
xintercept¶
xintercept is a special component that allows you to render a repeatable shape horizontally into another shape.
This is best described visually. Here we have an outline of a dala horse, and we are drawing rectangles within it.
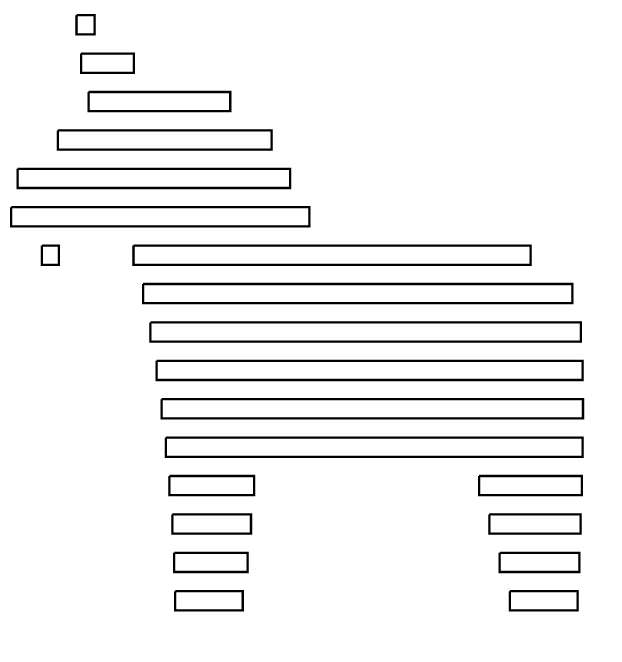
And here it is if the outline were drawn in.
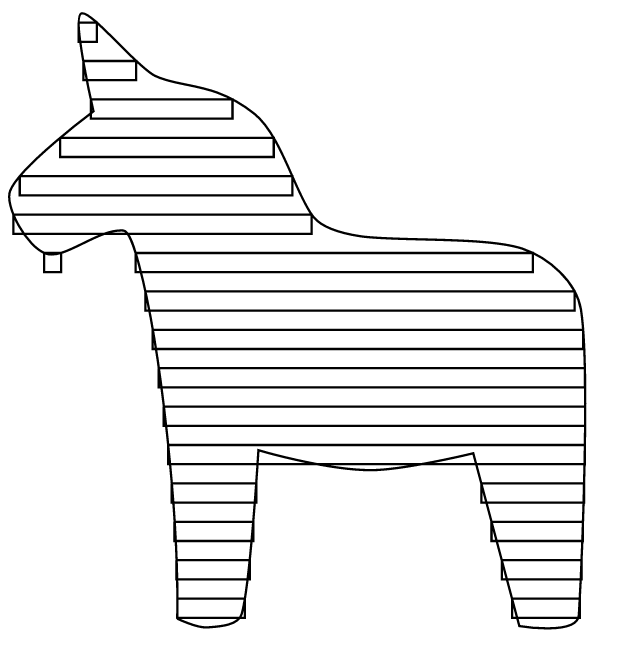
Parameters¶
outline
: <component> The shape that the repeatable should be drawn intorepeatable
: <component> The shape that should be repeated. The repeatableshape will have params passed down to allow it to render the proper size (see Local Variables).
initial_spacing
: how much initial vertical space to add before drawing the first repeatable.repeat_spacing
: how much vertical space between each repeatable.
Local Variables¶
xintercept__length
The horizontal length of this piecexintercept__to__x
The point to draw toxintercept__to__y
The point to draw toxintercept__from__x
The point to start fromxintercept__from__y
The point to start from
around¶
Examples
around is a component that allows you to render a repeatable shape N number of times around a circle.
Here we have a rectangle repeated nine times with a radius of 2.
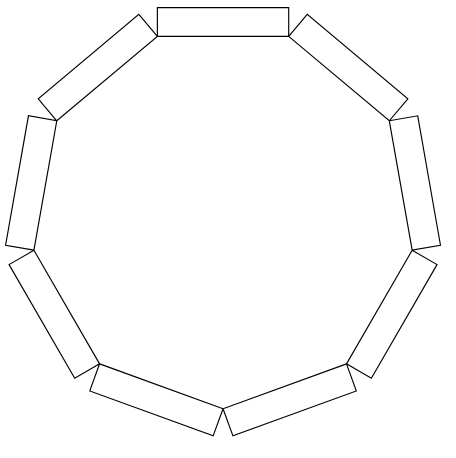
Parameters¶
center_point
: Where the center of the circle should berepeatable
: <component> The shape that should be repeated. The shape shouldbe rendered horizontally. The component will automatically move and rotate into the proper position. The repeatable shape will have params passed down to allow it to render the proper size. (see Local Variables)
num_edges
: the number of edges to draw. (i.e. 5 edges would be a pentagon)radius
: The radius of the circle. Note the start and end of the repeatable will be this distance from the center point
Local Variables¶
around__length
The horizontal length of this peicearound__index
The index of this piece. 0 to num_edges-1
gear¶
Examples
Renders a basic involute gear, suitable for most things. By default the Gear is rendered where 0,0 is the center point.
Parameters¶
teeth
: number of teeth on the geartooth_width
: the width of a single tooth.pressure_angle
: defaults to 20clearance
: defaults to 0.01backlash
: defaults to 0.01gear_variable_name
: if you set this name then certain Attributes of this gear will be available to all subsequently rendered components. See Global Variables
Global Variables¶
<gear_variable_name>__outer_radius
: The radius from center to tooth tip<gear_variable_name>__inner_radius
: The radius from center to lowest valley